The Importance of URL Escape Techniques in Web Development
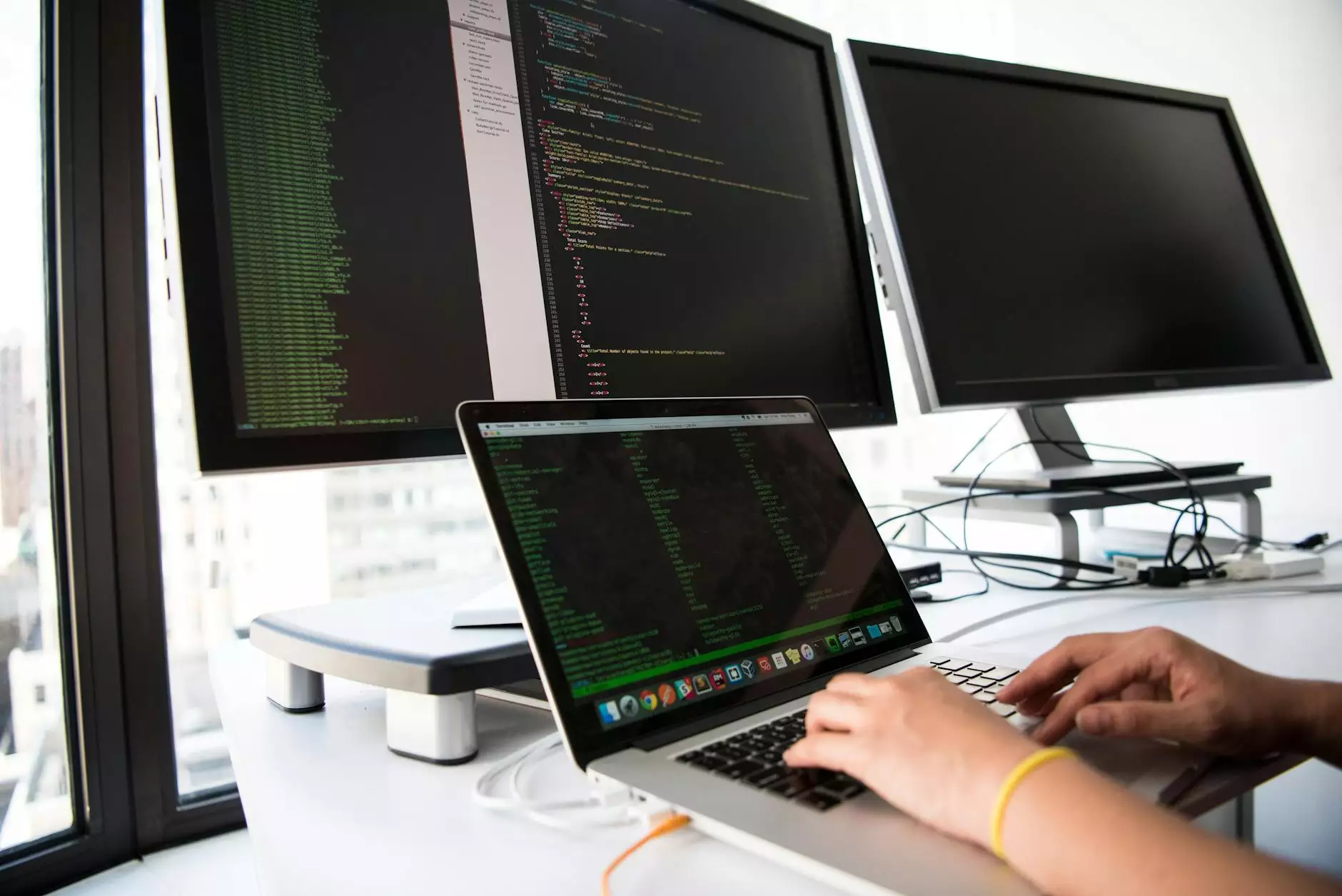
In the vast realm of web development, URL escape techniques play a pivotal role in ensuring that data is transmitted correctly over the internet. Given the growth of digital platforms and the increasing complexity of web applications, understanding how to encode special characters in a URL will not only enhance your web development skills but also improve the overall user experience on your website. In this article, we will explore what URL escaping entails, why it's essential, and how it can be effectively implemented in various programming languages.
Understanding URL Encoding
To appreciate URL escape, we first need to grasp the concept of URL encoding. URLs (Uniform Resource Locators) are the addresses used to access resources on the web. However, URLs can only be sent over the Internet using the ASCII character set. Therefore, characters outside this set need to be encoded. This is where URL encoding comes into play.
What is URL Encoding?
URL encoding, also known as percent encoding, is the process of replacing unsafe ASCII characters in a URL with a "%" followed by two hexadecimal digits. This technique serves to make URLs more compliant and helps in the correct interpretation of the different parts of a URL.
- Reserved characters: Characters like `?`, `&`, and `=` have special meanings in URLs. Encoding them prevents them from being misinterpreted.
- Unsafe characters: Characters such as spaces, `"`, ``, and others can cause issues if not encoded. For instance, a space character is represented as `%20` in a URL.
- Non-ASCII characters: Characters from languages other than English, such as Chinese or Arabic, must also be encoded to ensure proper transmission.
Why is URL Escape Essential?
The necessity of URL escape techniques cannot be overstated. Here are several reasons why these encoding techniques are crucial in web development:
1. Data Integrity and Security
When dealing with user input in web applications, failing to properly encode special characters can lead to security vulnerabilities, including Cross Site Scripting (XSS) and injection attacks. By applying URL encoding, developers can mitigate these risks significantly.
2. Improved User Experience
Well-formed URLs are much easier for users to read and understand. For instance, a URL like http://example.com/user?id=123 is far preferable to http://example.com/user?id=123%3Fuse%20invalid%20chars. Using proper URL escape techniques helps ensure that users have a smooth browsing experience.
3. Compatibility Across Different Browsers
Different browsers may interpret URLs differently, especially if they contain special characters. Using URL escape techniques ensures that your links work uniformly across various platforms, avoiding potential conflicts and errors.
Practical Applications of URL Escape in Different Programming Languages
Different programming languages provide various functions and libraries to handle URL escaping. Here we discuss how to implement URL escape in a few popular programming languages:
1. JavaScript
In JavaScript, you can utilize the encodeURIComponent() function to escape special characters in a URL.
let myString = "Hello World!"; let encodedString = encodeURIComponent(myString); console.log(encodedString); // Outputs: Hello%20World%212. Python
In Python, you can achieve URL escaping with the urllib.parse.quote() function.
import urllib.parse my_string = "Hello World!" encoded_string = urllib.parse.quote(my_string) print(encoded_string) # Outputs: Hello%20World%213. PHP
In PHP, the urlencode() function is the go-to method for escaping URLs.
$myString = "Hello World!"; $encodedString = urlencode($myString); echo $encodedString; // Outputs: Hello+World%214. Java
In Java, you can use the URLEncoder class to handle URL encoding:
import java.io.UnsupportedEncodingException; import java.net.URLEncoder; public class Main { public static void main(String[] args) throws UnsupportedEncodingException { String myString = "Hello World!"; String encodedString = URLEncoder.encode(myString, "UTF-8"); System.out.println(encodedString); // Outputs: Hello+World%21 } }Best Practices for URL Encoding
While understanding how to implement URL escape techniques is essential, it’s equally important to adhere to best practices to ensure consistent and efficient URL management:
- Always encode user input: Whenever you accept input from users and construct URLs dynamically, ensure that the data is encoded.
- Keep URLs as clean as possible: Avoid unnecessary encoding that could complicate the URL structure.
- Use descriptive names for parameters: This enhances readability and maintainability.
- Test links thoroughly: Regularly check your links for proper formatting and functionality, especially after updates to your site.
- Standardize your URL structure: Consistency in URL patterns aids in SEO and user experience.
Challenges with URL Escape
Despite its importance, implementing URL escape techniques comes with its challenges:
1. Misinterpretation of Encoded URLs
Some browsers may misinterpret encoded URLs, leading to unexpected behavior. Ensuring that you follow best practices can help mitigate these risks.
2. Debugging Complexity
Debugging applications with heavily encoded URLs can be challenging, making it sometimes hard to identify problems. A consistent coding style can aid in alleviating these difficulties.
3. Performance Considerations
While URL encoding is essential, unnecessary complexity in URLs might lead to performance issues. Always strive for optimized URL structures.
The Future of URL Escape Techniques
As web technologies continue to evolve, the importance of URL escape techniques will remain pivotal. With advancements in web frameworks and libraries, developers will have even more tools at their disposal to efficiently handle URL encoding and enhance web security.
Furthermore, as the Internet of Things (IoT) grows, understanding URL encoding will become increasingly important. Every device connected to the internet must communicate through URLs, making URL escape knowledge critical for developers in the future.
Conclusion
In conclusion, mastering URL escape techniques is not only beneficial but essential in the web development landscape. By ensuring the proper encoding of special characters within URLs, developers can create applications that are stable, secure, and user-friendly.
As you embark on your web development journey or look to deepen your knowledge, make URL escape a core part of your skill set. Emphasis on clean, well-structured URLs will not only enhance user experience but also contribute positively to your site’s search engine rankings.
For more information on web design and software development, visit semalt.tools and stay updated with best practices in the industry.